1 2 3 |
findQuestionById(id: String): Promise { return this.http.get("/question/" + id).toPromise(); } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
import { Component, OnInit } from "@angular/core"; import { ActivatedRoute } from "../../../../node_modules/@angular/router"; import { QuestionsService, CompositeQuestion } from "../../services/questions.service"; @Component({ selector: 'qm-body', templateUrl: './question.component.html' }) export class QuestionComponent implements OnInit { compositeQuestion: CompositeQuestion; questionId: string; constructor(private route: ActivatedRoute, private questionsService: QuestionsService) { } ngOnInit(): void { this.questionId = this.route.snapshot.params['id']; this.questionsService.findQuestionById(this.questionId).then(r => { this.compositeQuestion = r; }); } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
<div id="page-wrapper"> <div class="row"> <div class="col-lg-12"> <h1 class="page-header">Question</h1> </div> <!-- /.col-lg-12 --> </div> <div> <div class="row"> <div class="col-lg-12"> <div class="form-group"> <label>Question</label> <p class="form-control-static">{{ compositeQuestion.description }}</p> </div> <div class="form-group"> <label>Category</label> <p class="form-control-static">{{ compositeQuestion.category.name }}</p> </div> <div class="form-group"> <label>Options</label> <ul *ngFor="let option of compositeQuestion.options"> <li> {{ option.description }} | <a href="#"> Edit</a> | <a href="#"> Delete</a> </li> </ul> </div> <div class="form-group"> <button type="button" class="btn btn-success"><i class="fa fa-plus"></i> Add New Option</button> </div> </div> <!-- /.col-lg-12 --> </div> <!-- /.row --> </div> |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
import { NgModule } from "@angular/core"; import { CategoriesComponent } from "./categories/categories.component"; import { CommonModule } from "@angular/common"; import { FormsModule } from '@angular/forms'; import { AllQuestionsComponent } from "./all_questions/all_questions.component"; import { QuestionComponent } from "./all_questions/question.component"; import { AppRoutingModule } from "../app-routing.module"; @NgModule({ declarations: [ CategoriesComponent, AllQuestionsComponent, QuestionComponent ], imports: [ CommonModule, FormsModule, AppRoutingModule ], exports: [ CategoriesComponent, AllQuestionsComponent, QuestionComponent ] }) export class QuestionsModule { } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { DashboardComponent } from './dashboard/dashboard.component'; import { CategoriesComponent } from './questions/categories/categories.component'; import { AllQuestionsComponent } from './questions/all_questions/all_questions.component'; import { QuestionComponent } from './questions/all_questions/question.component'; const routes: Routes = [ { path: '', component: DashboardComponent }, { path: 'dashboard', component: DashboardComponent }, { path: 'categories', component: CategoriesComponent }, { path: 'questions', component: AllQuestionsComponent }, { path: 'question/:id', component: QuestionComponent }, { path: '**', pathMatch: 'full', redirectTo: '/' } ] @NgModule({ imports: [ RouterModule.forRoot(routes) ], exports: [ RouterModule ] }) export class AppRoutingModule { } |
1 |
<button type="button" class="btn btn-primary btn-circle"><i class="fa fa-eye"></i></button> |
1 |
<a class="btn-primary btn-circle fa fa-eye" routerLink="/question/{{ c.id }}"> </a> |
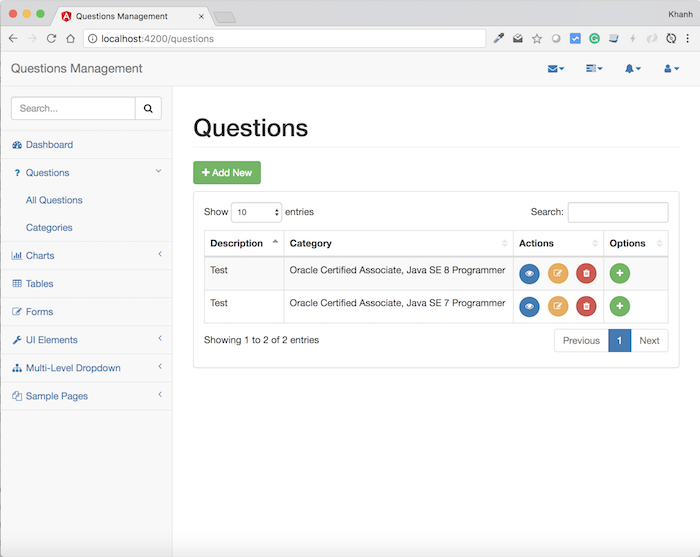
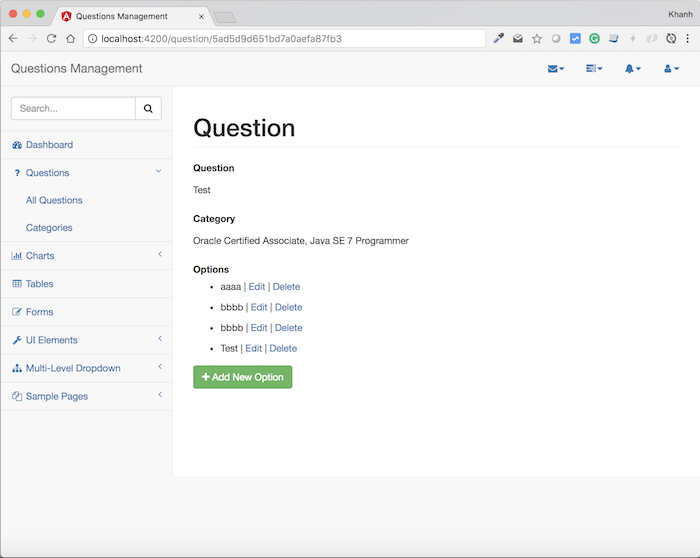